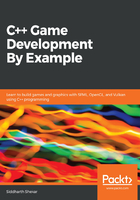
Statements
A program may not always be linear. Depending on your requirements, you might have to branch out or bifurcate, repeat a set of code, or take a decision. For this, there are conditional statements and loops.
In a conditional statement, you will check whether a condition is true. If it is, you will go ahead and execute the statement.
The first of the conditional statements is the if statement. The syntax for this looks as follows:
If (condition) statement;
Let's look at how to use this in code in the following code. Let's use one of the comparison operators here:
#include <iostream> #include <conio.h> // Program prints out values to screen int main() { int a = 36; int b = 5; if (a > b) std::cout << a << " is greater than " << b << std::endl; _getch(); return 0; }
The output is as follows:

We check the condition if a is greater than b, and if the condition is true, then we print out the statement.
But what if the opposite was true? For this, we have the if...else statement, which is a statement that basically executes the alternate statement. The syntax looks like this:
if (condition) statement1; else statement2;
Let's look at it in code:
#include <iostream> #include <conio.h> // Program prints out values to screen int main() { int a = 2; int b = 28; if (a > b) std::cout << a << " is greater than " << b << std::endl; else std::cout << b << " is greater than " << a << std::endl; _getch(); return 0; }
Here, the values of a and b are changed so that b is greater than a:

One thing to note is that after the if and else conditions, C++ will execute a single line of statement. If there are multiple statements after the if or else, then the statements need to be in curly brackets, as shown:
if (a > b) { std::cout << a << " is greater than " << b << std::endl; } else { std::cout << b << " is greater than " << a << std::endl; }
You can also have if statements after using else if:
#include <iostream> #include <conio.h> // Program prints out values to screen int main() { int a = 28; int b = 28; if (a > b) { std::cout << a << " is greater than " << b << std::endl; } else if (a == b) { std::cout << a << " is equal to " << b << std::endl; } else { std::cout << b << " is greater than " << a << std::endl; } _getch(); return 0; }
The output is as follows:
