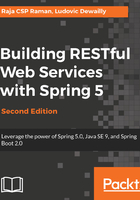
Sample values in the repository
In order to use the repository, we will have to create a concrete class and fill in some values to test the GET operation. In the following method, we can do that:
package com.packtpub.reactive;
// import statements
public class UserRepositorySample implements UserRepository {
// initiate Users
private final Map<Integer, User> users = new HashMap<>();
// fill dummy values for testing
public UserRepositorySample() {
this.users.put(100, new User(100, "David"));
this.users.put(101, new User(101, "John"));
this.users.put(102, new User(102, "Kevin"));
}
}
In the preceding class, we just implemented UserRepository and filled in some sample values.
In order to simplify our code, we have used only application-based data storage, which means that once the application is restarted, our data will be reinitialized. In this case, we can't store any new data in our application. However, this will help us to focus on our main topics, such as Reactive and Spring 5, which are not related to persistence.
We can use this sample repository in the routing method:
public RouterFunction<ServerResponse> routingFunction() {
UserRepository repository = new UserRepositorySample();
UserHandler handler = new UserHandler(repository);
}
The preceding lines will insert dummy values in our repository. This will be enough for testing the GET operation.