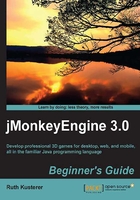
Time for action – rotate it again, Sam
Think of a quaternion as a Java object capable of storing rotations, which you can then apply to a geometry. Don't worry, the 3D engine does the tough calculation work for you!
As an exercise, let's create a Quaternion
object that accepts a 45-degree rotation around the x axis:
- Create a
Quaternion
object:Quaternion roll045 = new Quaternion();
- Supply the angle and the axis as arguments. The angle is
45*FastMath.DEG_TO_RAD
. The constant for the x axis isVector3f.UNIT_X
. This gives us:roll045.fromAngleAxis( 45*FastMath.DEG_TO_RAD , Vector3f.UNIT_X );
- Replace the relative
rotate()
method from the previous exercises and apply this absolute rotation instead:geom2.setLocalRotation( roll045 );
- Clean and build the
BasicGame
template, then right-click on it and run the file. - The yellow cube should be rotated by 45° as before.
What just happened?
To store a rotation in a com.jme3.math.Quaternion
object, you specify two values—the angle in radians, and the axis of the rotation.
The axis is defined by a type of vector called unit vector. A unit vector works just like any other vector; the only difference is that we do not care about its length (which is always 1 WU, hence the name). We only need the unit vector for its direction. Here are the most common examples of rotation axes:

Quaternions are worth knowing because they have many useful applications:
- You can concatenate several rotations into one
Quaternion
by using themult()
method, which is faster than doing transformations in several steps. Note that the order of rotations is non-commutative, so the order in which you multiply the quaternions makes a difference.Quaternion pitchAndRoll = pitch180.mult(roll180);
- Re-use
Quaternion
constants; give them meaningful names, such as roll90, pitch45, or yaw180, that remind you of what they do.
Tip
You can interpolate rotations between two quaternions using spherical linear interpolation (slerp). An interpolation lets you precisely fast-forward and rewind a complex rotation between its start and end points. Create two quaternions q1 and q2, for example, for a 40 degrees and a 50 degrees rotation around the x axis. Apply them with different interpolation values between 0.0f and 1.0f:
Quaternion q3 = new Quaternion(); q3.slerp(q1, q2, 0.5f); geom2.setLocalRotation(q3);